JSON (JavaScript Object Notation) is one of the most widely used data formats in modern web development, APIs, and databases. It’s lightweight, easy to read, and straightforward to work with. However, as with any data format, keeping your JSON properly formatted is key to avoiding errors and ensuring your code works smoothly.
One of the most common challenges developers face while working with JSON is formatting. If your JSON data is cluttered and unreadable, it can lead to debugging nightmares, especially in large projects. In this guide, we’ll explore various ways to format JSON within your code editor and keep your projects running efficiently.
Why Is Formatting JSON Important?
Before diving into the specifics of how to format JSON, let’s understand why it matters. Properly formatted JSON isn’t just for aesthetics; it plays a critical role in reducing bugs, improving collaboration, and making your code more maintainable. Here’s why:
- Readability: Well-structured JSON is easier to read and comprehend, even when you revisit the code after weeks or months.
- Debugging: Having a clear format helps you spot syntax errors quickly, which can save time when debugging.
- Collaboration: In team-based projects, properly formatted JSON ensures that all team members can work with the data seamlessly, without confusion.
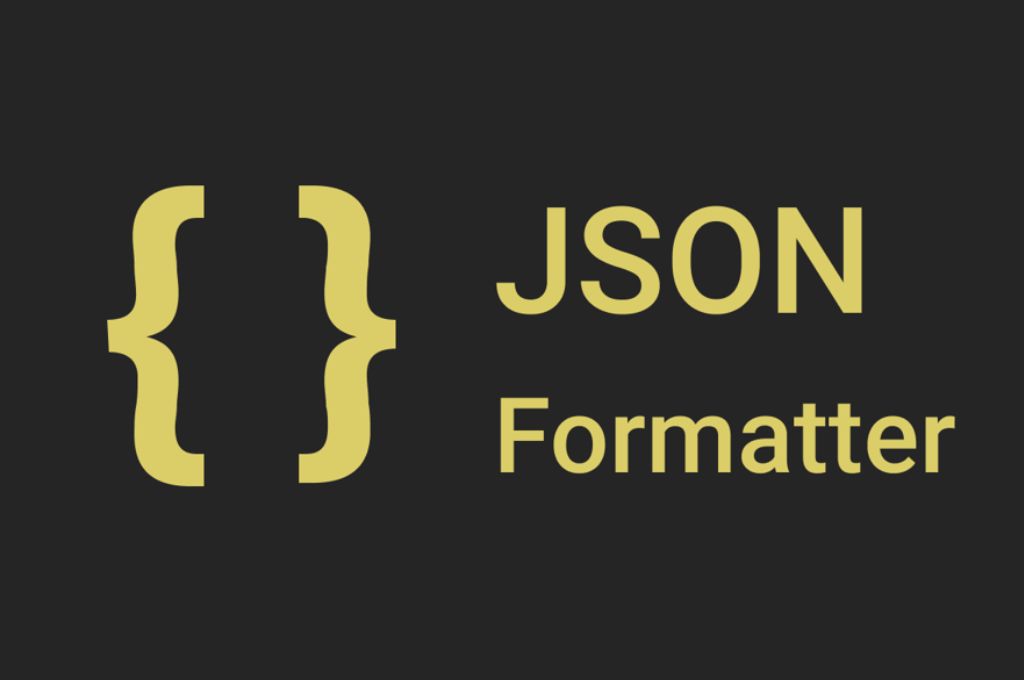
Common Challenges with JSON Formatting
1. Missing or Extra Commas
A common mistake when writing JSON is forgetting a comma between key-value pairs or adding an extra comma at the end. This results in syntax errors that can break your application.
Example of an error:
jsonCopy{
"name": "John",
"age": 30,
"city": "New York", <-- Extra comma here
}
2. Incorrect Braces or Brackets
Misplaced curly braces {}
or square brackets []
can cause parsing issues, making it hard to locate the error.
Example:
jsonCopy{
"name": "John",
"address": {
"street": "5th Avenue",
"city": "New York"
<-- Missing closing brace for the address object
}
3. Indentation Issues
JSON files can become hard to manage if proper indentation is ignored. Without consistent indentation, finding and fixing problems can become time-consuming, especially in larger files.
How to Format JSON Manually
If you’re working with small chunks of data or just want to make a quick fix, you might prefer manually formatting JSON. Here’s a basic guide:
Step 1: Ensure Correct Syntax
JSON syntax must include:
- Curly braces
{}
for objects. - Square brackets
[]
for arrays. - Key-value pairs separated by a colon
:
(e.g.,"name": "John"
). - Commas separating key-value pairs or elements within arrays, but no trailing comma at the end of an object or array.
Step 2: Add Indentation
For clarity, indent nested elements using 2 or 4 spaces (avoid tabs). For example:
Formatted JSON:
jsonCopy{
"name": "John",
"age": 30,
"address": {
"street": "5th Avenue",
"city": "New York"
}
}
Step 3: Ensure Key-Value Pair Consistency
Ensure every key has a corresponding value, and values are either strings, numbers, arrays, or objects.
Automated JSON Formatting Tools
While manual formatting is fine for small files, modern development requires efficient tools to format JSON quickly, especially when working with larger datasets. Thankfully, there are plenty of code editors and plugins that make this process seamless.
Popular Code Editors for Formatting JSON
Most code editors today come equipped with JSON formatting features. Here’s a look at some popular options:
Visual Studio Code (VS Code)
VS Code is one of the most popular code editors for web development, and it includes built-in support for JSON formatting.
Steps to Format JSON in VS Code:
- Open your JSON file.
- Select all the content using
Ctrl + A
(orCmd + A
on macOS). - Press
Shift + Alt + F
(Windows/Linux) orShift + Option + F
(macOS) to auto-format the file.
VS Code uses a default JSON formatter that aligns the code based on standard JSON conventions, including proper indentation and spacing.
Sublime Text
Sublime Text is another powerful code editor that supports JSON formatting, though it requires installing a package for auto-formatting.
Steps to Format JSON in Sublime Text:
- Install the Package Control package.
- Search for and install the Pretty JSON package.
- Once installed, select your JSON content and use the command
Ctrl + Shift + P
(Windows/Linux) orCmd + Shift + P
(macOS), then typePretty JSON: Format
.
This will instantly format your JSON file.
Atom
Atom is another widely used editor, and like VS Code, it comes with multiple options for formatting JSON. You can install packages such as atom-beautify
to enhance the formatting experience.
Steps to Format JSON in Atom:
- Install atom-beautify from the Atom package manager.
- Once installed, simply press
Ctrl + Alt + B
(orCmd + Alt + B
on macOS) to beautify your JSON file.
Online JSON Formatters
If you don’t want to install a code editor or need a quick solution, there are several online tools that can help you format your JSON data with ease.
1. JSONLint
JSONLint is one of the most popular online JSON validators and formatters. You can simply paste your raw JSON code, click on the “Validate JSON” button, and it will format and highlight any errors.
2. JSON Formatter & Validator
Another great option is JSON Formatter & Validator. This tool allows you to format, validate, and beautify your JSON data in a single click. You can also test how your JSON looks in a visual representation.
Conclusion
In summary, JSON formatting is essential to ensure your code is clean, maintainable, and error-free. Whether you’re formatting manually or using an automated tool, consistency is key. By leveraging the right tools and best practices, you can keep your JSON well-structured and easy to read.
Remember that well-formatted JSON isn’t just for debugging – it enhances the clarity of your code and allows teams to work more efficiently, even as your project grows. By following the steps outlined in this guide, you’ll avoid common pitfalls, streamline your workflow, and set yourself up for success in future projects.