In today’s digital world, data is at the heart of most applications and services. One of the most popular formats used to transmit and store data is JSON (JavaScript Object Notation). It is lightweight, easy to read, and language-independent, making it a preferred choice for developers, data scientists, and anyone working with APIs.
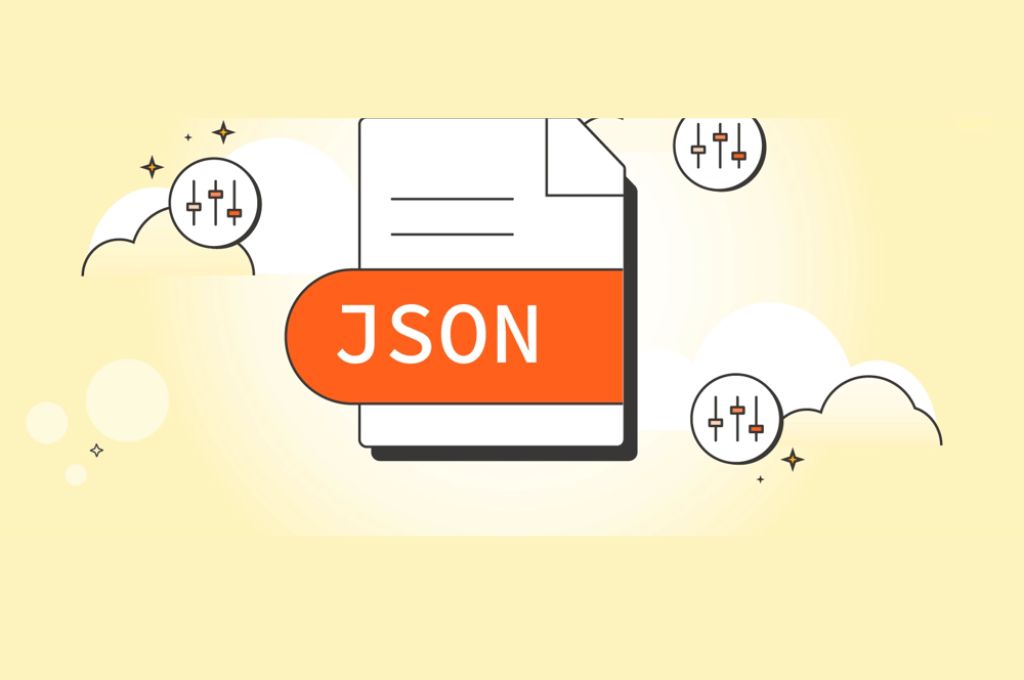
Why is JSON Formatting Important?
Proper formatting in JSON plays a crucial role in making data exchange more efficient and readable. Incorrectly formatted JSON can lead to errors, making it harder to debug and understand. As applications and systems scale, having a clear, consistent format ensures that developers and systems can easily read, process, and maintain data.
1. Consistent Indentation: Stick to One Style
One of the simplest, yet most overlooked aspects of JSON formatting is indentation. When JSON data grows, nested objects and arrays can become difficult to read without proper indentation. The common rule of thumb is to use two or four spaces for indentation.
Expert Tip:
JSON parsers ignore spaces and indentation, but maintaining consistency in indentation styles ensures that the data is easily readable by humans. This becomes critical when multiple developers are collaborating on the same project. JSON linting tools like jsonlint can help identify formatting issues.
Example of Proper Indentation:
jsonCopy{
"user": {
"name": "John Doe",
"age": 30,
"address": {
"street": "123 Elm St",
"city": "Sample Town"
}
}
}
2. Use Double Quotes for Keys and String Values
In JSON, both keys and string values must always be enclosed in double quotes (” “). Although some programming languages allow single quotes for strings, JSON strictly requires double quotes. Using single quotes or omitting quotes altogether will result in errors.
Expert Tip:
It’s not just a syntax issue; sticking to double quotes ensures your JSON data is valid across different systems and applications. The standardization improves code interoperability.
Correct JSON Example:
jsonCopy{
"name": "Jane",
"age": 25
}
3. Avoid Trailing Commas
Many developers may be tempted to add a comma after the last element in an object or array, especially when adding new items. However, trailing commas are not allowed in JSON syntax. Omitting this can prevent unnecessary parsing errors.
Expert Insight:
Trailing commas can be a source of frustrating bugs, especially when working in teams. As JSON data grows, it’s easy to overlook them, which can lead to tricky errors that are hard to debug.
Incorrect JSON Example:
jsonCopy{
"name": "Alice",
"age": 28, // Incorrect trailing comma
}
Correct JSON Example:
jsonCopy{
"name": "Alice",
"age": 28
}
4. Maintain Proper Nesting
When your JSON data contains nested objects or arrays, it’s important to ensure proper nesting. Each object should be clearly structured to make it easy for someone reading the code to trace its hierarchy.
Expert Insight:
Consider the data hierarchy and structure it logically. Too much nesting can lead to confusion and performance issues when parsing large datasets. If a structure is too complex, consider breaking it down into smaller, manageable components.
Example of Nested JSON:
jsonCopy{
"user": {
"name": "Emily",
"preferences": {
"theme": "dark",
"notifications": true
}
}
}
5. Use Meaningful Key Names
In JSON, keys serve as identifiers for data. Using clear, descriptive names helps other developers quickly understand the purpose of each field. Avoid using vague or single-letter keys like "a"
or "x"
unless it’s contextually clear.
Expert Insight:
Using descriptive keys helps not only with readability but also with long-term maintainability. Remember, someone else might be working on your code months or years after you’ve written it, so clarity is critical.
Bad Key Names Example:
jsonCopy{
"a": "John",
"x": "Doe"
}
Good Key Names Example:
jsonCopy{
"firstName": "John",
"lastName": "Doe"
}
6. Prefer Arrays Over Objects for Homogeneous Data
When dealing with lists of items that are similar in nature, such as a list of user names, arrays are the better choice over objects. Arrays provide a cleaner, more natural way to represent ordered data.
Expert Insight:
Using arrays helps reduce redundancy in your JSON data. It also simplifies parsing, making your data more flexible for consumption by APIs and other services.
Example of Array for Similar Data:
jsonCopy{
"users": ["John", "Jane", "Emily"]
}
Example of Incorrect Object Use:
jsonCopy{
"user1": "John",
"user2": "Jane",
"user3": "Emily"
}
7. Avoid Redundancy: Use References Instead
Sometimes, the same data appears multiple times in a JSON object. To optimize your JSON and avoid redundancy, use references. Instead of repeating the same data over and over, consider referencing it where needed.
Expert Insight:
By minimizing redundancy, you not only reduce the size of your data but also make it more maintainable. This is especially useful when dealing with large data structures or databases.
Example with Redundancy:
jsonCopy{
"author": "John Smith",
"book": "JavaScript Essentials",
"author_details": {
"name": "John Smith",
"birth_date": "1990-01-01"
}
}
Optimized Example Using References:
jsonCopy{
"author": "John Smith",
"book": "JavaScript Essentials",
"author_details": {
"$ref": "authors/1"
}
}
8. Validate Your JSON
Always validate your JSON data using a JSON validator tool to ensure there are no syntax errors. Tools like JSONLint or built-in validation in code editors like VSCode can help identify issues early.
Expert Insight:
Validating your JSON will save you from debugging hours later. It also ensures the integrity of the data you send or receive, especially when working with APIs or complex applications.
9. Version Your JSON Data Format
As your application grows and evolves, the structure of your JSON data may need to change. To manage this effectively, version control your JSON format. Add versioning information either in the file name or as a key within the data structure.
Expert Insight:
Versioning prevents conflicts when updating your data schema. It allows backward compatibility, which is essential when different versions of your application are using different data formats.
Example:
jsonCopy{
"version": "1.0",
"user": {
"name": "Sarah",
"age": 35
}
}
Conclusion
In conclusion, following best practices for JSON formatting can make a big difference in your development process. Proper indentation, clear key names, avoiding redundancy, and ensuring valid syntax will not only make your JSON data easier to read but also optimize its performance. Remember, the best way to ensure your data is structured correctly is by following a set of standards that promote consistency, clarity, and scalability.
By following these expert insights, you’ll build more maintainable, error-free JSON data that works efficiently for you and your team. Happy coding!